density_clustering.hpp File Reference
Density-based clustering. More...
#include <vector>
#include <map>
#include <set>
#include <array>
#include <utility>
#include <string>
#include <boost/program_options.hpp>
#include "tools.hpp"
Include dependency graph for density_clustering.hpp:
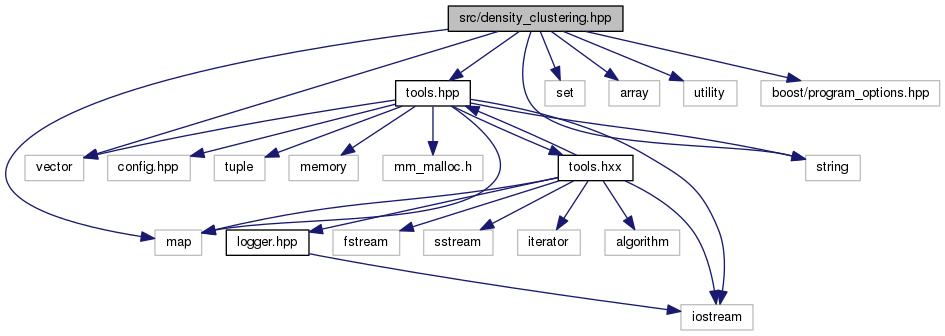
This graph shows which files directly or indirectly include this file:
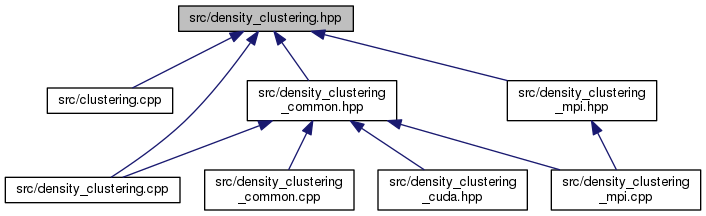
Go to the source code of this file.
Classes | |
struct | Clustering::Density::BoxGrid |
Namespaces | |
Clustering | |
general namespace for clustering package | |
Clustering::Density | |
namespace for density-based clustering functions | |
Typedefs | |
using | Clustering::Density::FreeEnergy = std::pair< std::size_t, float > |
matches frame id to free energy | |
using | Clustering::Density::Neighbor = Clustering::Tools::Neighbor |
matches neighbor's frame id to distance | |
using | Clustering::Density::Neighborhood = Clustering::Tools::Neighborhood |
map frame id to neighbors | |
using | Clustering::Density::Box = std::array< int, 2 > |
encodes 2D box for box-assisted search algorithm | |
Functions | |
constexpr Box | Clustering::Density::neighbor_box (const Box center, const int i_neighbor) |
BoxGrid | Clustering::Density::compute_box_grid (const float *coords, const std::size_t n_rows, const std::size_t n_cols, const float radius) |
bool | Clustering::Density::is_valid_box (const Box box, const BoxGrid &grid) |
std::vector< std::size_t > | Clustering::Density::calculate_populations (const float *coords, const std::size_t n_rows, const std::size_t n_cols, const float radius) |
calculate population of n-dimensional hypersphere per frame for one fixed radius. | |
std::map< float, std::vector< std::size_t > > | Clustering::Density::calculate_populations (const float *coords, const std::size_t n_rows, const std::size_t n_cols, std::vector< float > radii) |
std::vector< float > | Clustering::Density::calculate_free_energies (const std::vector< std::size_t > &pops) |
std::vector< FreeEnergy > | Clustering::Density::sorted_free_energies (const std::vector< float > &fe) |
std::tuple< Neighborhood, Neighborhood > | Clustering::Density::nearest_neighbors (const float *coords, const std::size_t n_rows, const std::size_t n_cols, const std::vector< float > &free_energy) |
void | Clustering::Density::screening_log (const double sigma2, const std::size_t first_frame_above_threshold, const std::vector< FreeEnergy > &fe_sorted) |
log output for screening steps | |
std::tuple< std::vector< std::size_t >, std::size_t, double, std::vector< FreeEnergy >, std::set< std::size_t >, std::size_t > | Clustering::Density::prepare_initial_clustering (const std::vector< float > &free_energy, const Neighborhood &nh, const float free_energy_threshold, const std::size_t n_rows, const std::vector< std::size_t > initial_clusters) |
std::vector< std::size_t > | Clustering::Density::normalized_cluster_names (std::size_t first_frame_above_threshold, std::vector< std::size_t > clustering, std::vector< FreeEnergy > &fe_sorted) |
return clustered trajectory with new, distinct cluster names. | |
bool | Clustering::Density::lump_initial_clusters (const std::set< std::size_t > &local_nh, std::size_t &distinct_name, std::vector< std::size_t > &clustering, const std::vector< FreeEnergy > &fe_sorted, std::size_t first_frame_above_threshold) |
lump clusters based on distance threshold in screening process | |
std::set< std::size_t > | Clustering::Density::high_density_neighborhood (const float *coords, const std::size_t n_cols, const std::vector< FreeEnergy > &sorted_fe, const std::size_t i_frame, const std::size_t limit, const float max_dist) |
double | Clustering::Density::compute_sigma2 (const Neighborhood &nh) |
bool | Clustering::Density::compare2DVector (const std::pair< std::size_t, std::size_t > &p1, const std::pair< std::size_t, std::size_t > &p2) |
compare two two dimensional pairs by their second entry | |
bool | Clustering::Density::has2digits (float val) |
check if float has at maximum two digits | |
std::vector< std::size_t > | Clustering::Density::sorted_cluster_names (std::vector< std::size_t > clustering) |
sorts the cluster by decreasing population and renames them from 1..N | |
std::vector< std::size_t > | Clustering::Density::assign_low_density_frames (const std::vector< std::size_t > &initial_clustering, const Neighborhood &nh_high_dens, const std::vector< float > &free_energy) |
void | Clustering::Density::main (boost::program_options::variables_map args) |
user interface and controlling function for density-based geometric clustering. More... | |
Variables | |
constexpr int | Clustering::Density::BOX_DIFF [9][2] |
const int | Clustering::Density::N_NEIGHBOR_BOXES = 9 |
number of neigbor boxes in 2D grid (including center box). | |
Detailed Description
Class Documentation
◆ Clustering::Density::BoxGrid
struct Clustering::Density::BoxGrid |
the full grid constructed for boxed-assisted nearest neighbor search with fixed distance criterion.
Definition at line 85 of file density_clustering.hpp.
Class Members | ||
---|---|---|
vector< Box > | assigned_box | matching frame id to the frame's assigned box |
map< Box, vector< int > > | boxes | the boxes with a list of assigned frame ids |
vector< int > | n_boxes | total number of boxes |