tools.hpp File Reference
Tools mainly for IO and some other functions. More...
#include "config.hpp"
#include <string>
#include <vector>
#include <map>
#include <tuple>
#include <memory>
#include <iostream>
#include <mm_malloc.h>
#include "tools.hxx"
Include dependency graph for tools.hpp:
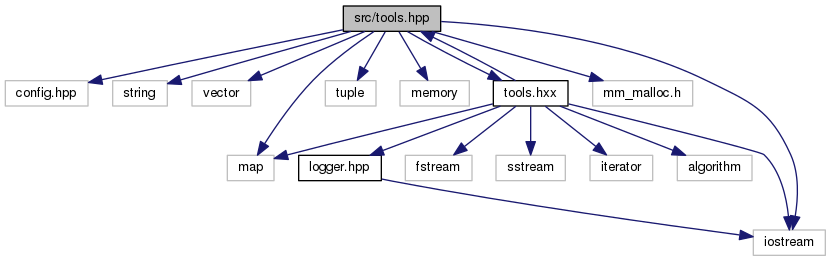
This graph shows which files directly or indirectly include this file:
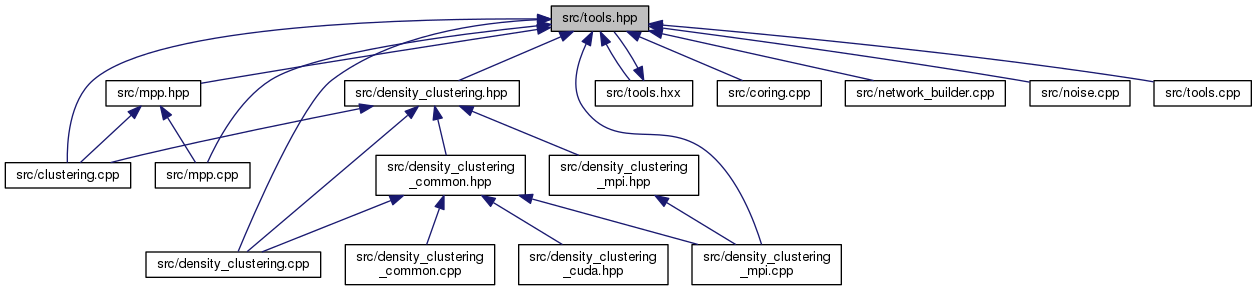
Go to the source code of this file.
Namespaces | |
Clustering | |
general namespace for clustering package | |
Clustering::Tools | |
additional tools used throughout the clustering package | |
Macros | |
#define | ASSUME_ALIGNED(c) (c) = (float*) __builtin_assume_aligned( (c), DC_MEM_ALIGNMENT) |
needed for aligned memory allocation for Xeon Phi, SSE or AVX | |
Typedefs | |
using | Clustering::Tools::Neighbor = std::pair< std::size_t, float > |
matches neighbor's frame id to distance | |
using | Clustering::Tools::Neighborhood = std::map< std::size_t, Clustering::Tools::Neighbor > |
map frame id to neighbors | |
Functions | |
void | Clustering::Tools::write_pops (std::string fname, std::vector< std::size_t > pops, std::string header_comment, std::map< std::string, float > stringMap) |
write populations as column into given file | |
void | Clustering::Tools::write_fes (std::string fname, std::vector< float > fes, std::string header_comment, std::map< std::string, float > stringMap) |
write free energies as column into given file | |
std::vector< std::size_t > | Clustering::Tools::read_clustered_trajectory (std::string filename) |
read states from trajectory (given as plain text file) | |
void | Clustering::Tools::write_clustered_trajectory (std::string filename, std::vector< std::size_t > traj, std::string header_comment, std::map< std::string, float > stringMap) |
write state trajectory into plain text file | |
template<typename NUM > | |
std::vector< NUM > | Clustering::Tools::read_single_column (std::string filename) |
read single column of numbers from given file. number type (int, float, ...) given as template parameter | |
template<typename NUM > | |
void | Clustering::Tools::write_single_column (std::string filename, std::vector< NUM > dat, std::string header_comment, bool with_scientific_format=false) |
write single column of numbers to given file. number type (int, float, ...) given as template parameter | |
template<typename KEY , typename VAL > | |
void | Clustering::Tools::write_map (std::string filename, std::map< KEY, VAL > mapping, std::string header_comment, bool val_then_key=false) |
write key-value map to plain text file with key as first and value as second column | |
std::vector< float > | Clustering::Tools::read_free_energies (std::string filename) |
read free energies from plain text file | |
std::vector< std::size_t > | Clustering::Tools::read_concat_limits (std::string filename) |
std::pair< Neighborhood, Neighborhood > | Clustering::Tools::read_neighborhood (const std::string filename) |
void | Clustering::Tools::write_neighborhood (const std::string filename, const Neighborhood &nh, const Neighborhood &nh_high_dens, std::string header_comment, std::map< std::string, float > stringMap) |
std::map< std::size_t, std::size_t > | Clustering::Tools::microstate_populations (std::vector< std::size_t > traj) |
compute microstate populations from clustered trajectory | |
template<typename NUM > | |
std::tuple< NUM *, std::size_t, std::size_t > | Clustering::Tools::read_coords (std::string filename, std::vector< std::size_t > usecols=std::vector< std::size_t >()) |
template<typename NUM > | |
void | Clustering::Tools::free_coords (NUM *coords) |
free memory pointing to coordinates | |
template<typename NUM > | |
std::vector< NUM > | Clustering::Tools::dim1_sorted_coords (const NUM *coords, std::size_t n_rows, std::size_t n_cols) |
template<typename NUM > | |
std::vector< NUM > | Clustering::Tools::boxlimits (const std::vector< NUM > &xs, std::size_t boxsize, std::size_t n_rows, std::size_t n_cols) |
template<typename NUM > | |
std::pair< std::size_t, std::size_t > | Clustering::Tools::min_max_box (const std::vector< NUM > &limits, NUM val, NUM radius) |
return indices of min and max boxes around value for given radius. | |
unsigned int | Clustering::Tools::min_multiplicator (unsigned int orig, unsigned int mult) |
return minimum multiplicator to fulfill result * mult >= orig | |
std::string | Clustering::Tools::stringprintf (const std::string &str,...) |
printf-version for std::string More... | |
template<typename NUM > | |
NUM | Clustering::Tools::string_to_num (const std::string &s) |
convert std::string to number of given template format | |
template<typename T > | |
std::vector< T > | Clustering::Tools::unique_elements (std::vector< T > xs) |
return distinct elements of vector | |
void | Clustering::Tools::check_concat_limits (std::vector< std::size_t > concat_limits, std::size_t n_frames) |
check if concat limits were passed correctly | |
void | Clustering::Tools::read_comments (std::string filename, std::map< std::string, float > &stringMap) |
read comments of stringMap from file. Comments should start with #@ | |
float | Clustering::Tools::read_next_float (std::ifstream &ifs) |
read the next float of ifstream | |
void | Clustering::Tools::append_commentsMap (std::string &header_comment, std::map< std::string, float > &stringMap) |
append commentsMap to header comment | |
Detailed Description
Tools mainly for IO and some other functions.
- See also
- Clustering::Tools
Definition in file tools.hpp.